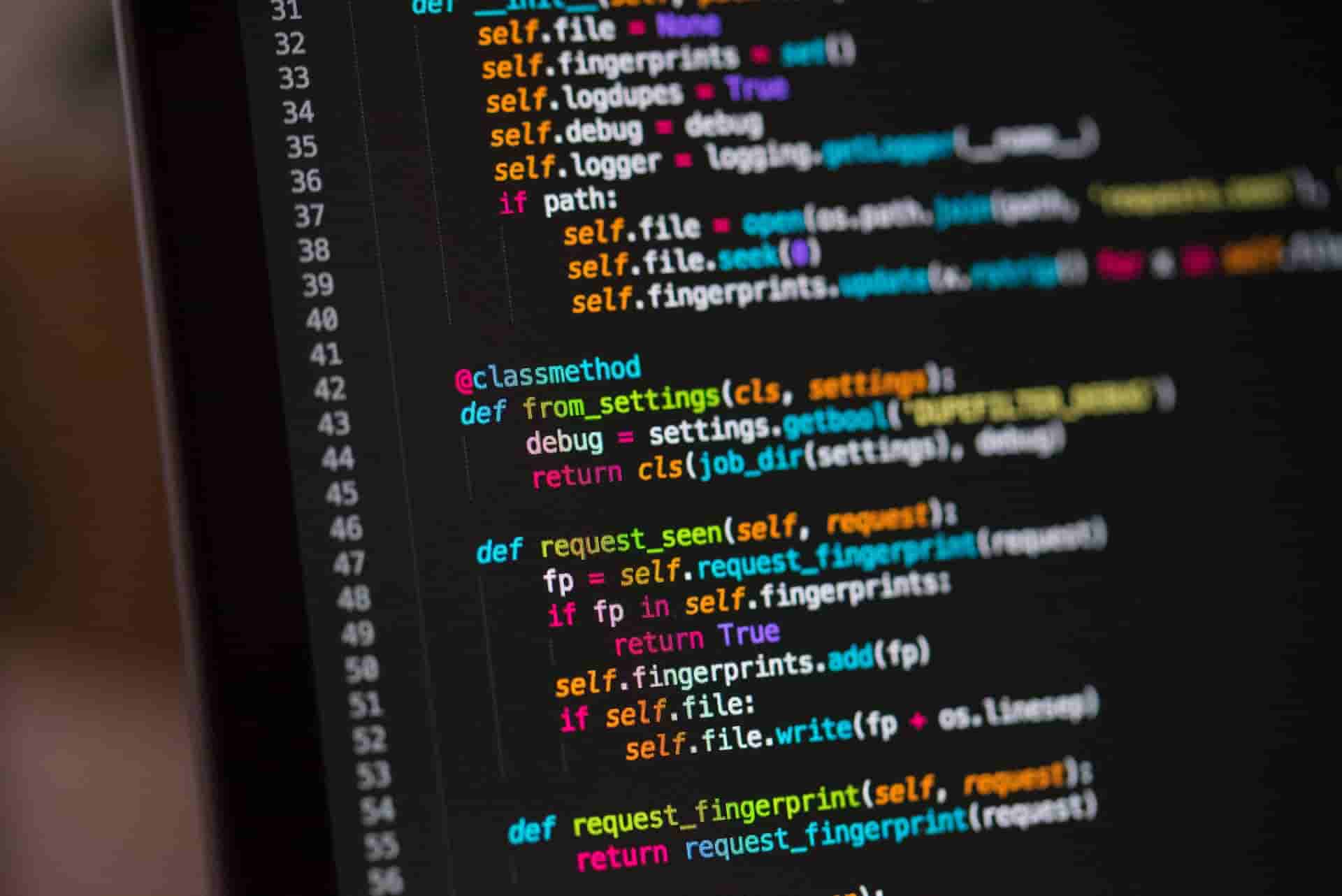
Anyone can code Learn the Basics: Logic and Branches
For anyone unaware, this is part 2 in an introductory series in the basics of programming - if you haven’t already I’d strongly reccomend going back and reading the previous blog post here… For the rest of you, let us continue.
Today we will be tackling the basics of logic, enough for you to be able to understand branch instructions in programming. Logic is a fairly deep topic that trips a lot of us up, I am not intending to explain this philosophically - that would take far too long. Instead I intend to explain it from a computer science perspective but as simply as possible.
Logic for Computers
Computers are fundamentally logic machines, they deal entirely in 1’s and 0’s (otherwise interpreted as Trues and Falses). That is to say that 1 == True
and 0 == False
(I will explain my use of double equals later - for now it means: the same as). In order to make something capable of doing anything with these two very basic things (True and False), we must have operators over these things that allow us to manipulate them. What is an operator? An operator in the logical context is exactly the same as an operator for a number, the number operators are: + = × ÷ etc. These operators are binary - that is they affect two numbers at once (you may be able to write the sum 1+2+3 but thats technically (1+2)+3 i.e. you add 1 and 2 first and then add that result to 3).
Ok now you understand what an operator is, I can tell you what some of the logical operators are. You will only need 3 to do every logical operation possible - there are more but they’re essentially just there for easy reading and are not needed for this guide. The ones we are interested in are AND, OR, and NOT, these each do different things to our Trues and Falses.
AND
AND gives the result True only if both values surrounding it are True (the values surrounding it are whats called the inputs - so for the sum: 1 + 2 the inputs to the operator “+” would be “1” and “2”). An example of this would be:
True AND True
TrueTrue AND False
False
Try heading to IPython again and messing around with True and False. Before you go however I’ll need to tell you how to do this in Python. In Python you must type True exactly like I just have, i.e. it must have a capital T, False must also have a capital F. Furthermore, in this guide I have and will continue to capitalise AND, OR, and NOT to distinguish them from the regular words, in Python you simply write these in the lower case. So for the first example above you would write True and True
instead, hopefully that’s clear. If you manage to get any of that wrong Python will give you a syntax error (basically a wording/grammar error for computers).
Back? Ok, hopefully you managed to replicate my examples above and get the same results. Don’t worry if you accidentally put in a string or integer instead of True or False and got some unexpected results. Python can also use AND, OR, and NOT on many other data types but that is beyond the scope of this guide.
OR
OR gives the result True if either of the two inputs is True, i.e. it only ever gives False if both inputs are False, think of it like the opposite of AND. Again, examples below:
True OR True
TrueTrue OR False
TrueFalse OR False
False
Again, I’d recommend heading back to IPython to try out these examples for yourself, remember what I said earlier however.
NOT
NOT is slightly different as it only takes one input (not binary like AND and OR). It simply inverts its single input, so if you applied it to True it would give you False and if you applied it to False it would give you True. I can only show two examples here so I might as well show both:
NOT True
FalseNOT False
True
Once again, try this in IPython remembering what I said before in the AND section.
Combining Logical Operators
As you might have guessed you can combine these operators to create more complex logic, remember BIDMAS? Just a quick refresher, BIDMAS is the precedence of the mathematical operators - this simply means their priority. If you had the sum 1 + 2 × 3
then you’d have to multiply 2 and 3 together first and then add the 1, even though the section of the sum 1 + 2
is furthest to the left. The precedence of the logical operators we have talked about (AND, OR, and NOT) is in the order NOT → AND → OR (with NOT having the highest priority and OR having the lowest). I’ll put a couple of examples below (with workings):
NOT True OR True AND True
False OR True AND True
False OR True
TrueNOT False AND NOT False OR False
True AND True OR False
True OR False
True
Now that we’ve dealt with logic we can move on to branching instructions, be sure to go back and refresh this if you get stuck later.
Branching Instructions
A branch instruction is pretty self explanatory, it is an instruction (a bit like a step in a cook-book recipe) that can take the program execution down a different path (or determine which set of cook book instructions you take). There are a few types, however I will cover If and Else statements here.
An If statement only executes the code within its body (something’s body means its contents) if its condition is True, see why we did logic first? So for example you could have the code:
if True:
print("This should print")
This should print
Notice the “print” in the code above? That’s a function (these will be explained in a later post) that puts the text within it onto the screen. You can try typing this into IPython, in order to type a new line without IPython executing the code straight away press shift + Enter you’ll then need to press Enter twice again after the last line in the body of the if statement in order to execute it.
Another thing I should probably explain is the indentation, in Python we use indentation to tell Python that something is contained within something else. In this case the line print("This should print")
is within the If statement, that means that it will only ever exectute if the If statement ends up being True (which is the case for the above example). If we were to write the line print("This should print")
in the same place but not indented it would always print regardless of whether the If statement evaluated to be True.
If you were to swap the “True” with a “False” in the example above what do you think would happen? Well nothing, actually, the computer would read “if False” and decide not to execute the body and therefore not print anything to the screen.
This alone may seem pretty useless, how are you going to do anything with this since you already know the “True” or “False” value? The answer is that we don’t ever use True or False as the condition for an if statement, instead we use an expression that evaluates to either True or False. What does that mean? Well, if I were to tell you that 1 was equal to 2 you would tell me that I’m an idiot and that that’s totally wrong. Computers are beings of few words however and would simply reply with “False”, this in turn could be used in an if statement to find something out. That previous expression translated to Python would look like 1 == 2
which would evaluate to “False”, if we used that in conjunction with an if statement we could construct (the double equals means check if these two things are the same - if so replace the whole thing with True, if not False):
if 1 == 2:
print("You have just broken maths")
Again, notice that there’s no output here, 1 does not equal 2 therefore the expression 1 == 2
would evaluate to “False” and “if False” never executes meaning the print statement isn’t executed either.
This same logic can be used when evaluating if one word is equal to another, in Python we represent a string (again, fancy programmer terminology for word/sentence) using quotes (can be either double quotes: “” or single quotes: ‘’ that wrap the word/sentence we want to be the string “like this” or ‘like this’ - both are valid). See example below:
if "this sentence/word" == "this sentence/word":
print("This should print")
This should print
Else
One thing I haven’t touched on yet is the Else statement, this is only used in conjunction with If to allow the program to do one thing for one condition or do another thing if that condition ends up being False. If you don’t use an Else then the next line underneath the If statement will be executed whether the If statement evaluates to True or not. You can think of this as: IF something, do this, ELSE, do something else. You don’t need to use Else, if you don’t the computer will compute “IF something, do this” and only execute “this” if the “something” turns out to be True, it will then read the next instruction on the next line beneath it. Maybe an example will clear this up:
if "this sentence/word" == "this sentence/word":
print("This should print")
else:
print("This should not print")
print("This should always print")
This should print
This should always print
Putting it all together
We can combine these types of “the same as” expressions with the formal logical operators we went over earlier. Since the expression 1 == 1
will be evaluated and seen by the computer as “True” and the expression 1 == 2
will be seen as “False” we can simply combine these with ease. For example we could use OR to combine those two expressions into one single expression (let’s say you wanted some code to be executed if either of two conditions were met). The way we would represent that is as follows:
if 1 == 1 OR 1 == 2:
print("This should print")
else:
print("This should not print")
print("This should always print")
This should print
This should always print
Remember, I am using capitalised “OR” here to make it obvious that it’s the operator not the word, when writing Python code you would simply write OR in the lower case. Be sure to experiment with these examples in IPython to help you fully grasp all these concepts.
This may seem difficult at first but it’s just got to click, keep at it and you’ll do fine. Again, if I have overexplained or underexplained let me know, feedback is always welcome - email below :)